This example demonstrate wait and notify example. Main thread(ThreadA) will create threadB and will start threadB. After threadB started, it just print that it is started and will go to WAITING state by calling wait(). Meanwhile threadA goes to sleep for 3 seconds and will print that it is awaked and will notify threadB by calling notify(). This will cause to threadB goes to RUNNABLE state. Then it will resume the threadB's execution and will print that it is notified.
Note that when we call wait() and notify(), it should call inside synchronised context. Otherwise it will throw java.lang.IllegalMonitorStateException. We have to pass a lock object to the synchronised block. That object will be blocked during the execution of synchronisation block. In this case I pass the threadB itself as the lock object.
Tuesday, February 28, 2017
Subscribe to:
Posts (Atom)
How to choose IP address range for resources for AWS virtual private cloud
You will often need to allocate an IP address range when you design the network of an AWS VPC. Since VPC is a small network of resources(E...
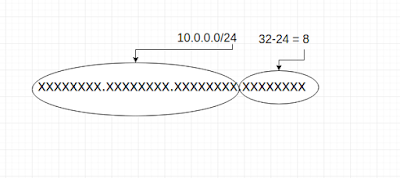
-
You can create OAuth applications using OAuthAdminService admin service. You can follow the below steps to see how mapping of existing OAu...
-
It can be white listed and black listed applications in WSO2 EMM 2.1.0 onwards. What is Application White List? White listed applications...
-
JRE will create and update a file in a directory called oracle_jre_usage located in Windows : %ProgramData%\Oracle\Java\.oracle_jre-usa...